Plot saccadic main sequence#
In this notebook we show how you can load a dataset, compute all the necessary properties and the plot the main sequence.
What you will learn in this tutorial:#
how to prepare your data to plot the saccadic main sequence
how to create a main sequence plot of your saccade events and style it to your liking
Loading and preprocessing your data#
We import pymovements
as the alias pm
for convenience.
[1]:
import pymovements as pm
/home/docs/checkouts/readthedocs.org/user_builds/pymovements/envs/stable/lib/python3.9/site-packages/tqdm/auto.py:21: TqdmWarning: IProgress not found. Please update jupyter and ipywidgets. See https://ipywidgets.readthedocs.io/en/stable/user_install.html
from .autonotebook import tqdm as notebook_tqdm
Let’s start by downloading our ToyDataset
and loading in its data:
[2]:
dataset = pm.Dataset('ToyDataset', path='data/ToyDataset')
dataset.download()
dataset.load()
Using already downloaded and verified file: data/ToyDataset/downloads/pymovements-toy-dataset.zip
Extracting pymovements-toy-dataset.zip to data/ToyDataset/raw
100%|██████████| 20/20 [00:00<00:00, 20.61it/s]
[2]:
<pymovements.dataset.dataset.Dataset at 0x7f46665608b0>
Now, you have to convert the raw x and y coordinates in pixels to degrees in visual angle.
[3]:
dataset.pix2deg()
100%|██████████| 20/20 [00:01<00:00, 10.61it/s]
[3]:
<pymovements.dataset.dataset.Dataset at 0x7f46665608b0>
Next we can convert these positions into velocitites.
[4]:
dataset.pos2vel()
100%|██████████| 20/20 [00:01<00:00, 19.35it/s]
[4]:
<pymovements.dataset.dataset.Dataset at 0x7f46665608b0>
Let’s check if we now have all our expected columns:
[5]:
dataset.gaze[0].frame.head()
[5]:
time | stimuli_x | stimuli_y | text_id | page_id | pixel | position | velocity |
---|---|---|---|---|---|---|---|
f32 | f32 | f32 | i64 | i64 | list[f32] | list[f32] | list[f32] |
1.988145e6 | -1.0 | -1.0 | 0 | 1 | [206.800003, 152.399994] | [-10.697598, -8.8524] | [null, null] |
1.988146e6 | -1.0 | -1.0 | 0 | 1 | [206.899994, 152.100006] | [-10.695184, -8.859678] | [null, null] |
1.988147e6 | -1.0 | -1.0 | 0 | 1 | [207.0, 151.800003] | [-10.692768, -8.866957] | [1.610438, -5.256017] |
1.988148e6 | -1.0 | -1.0 | 0 | 1 | [207.100006, 151.699997] | [-10.690351, -8.869382] | [0.40261, -4.447301] |
1.988149e6 | -1.0 | -1.0 | 0 | 1 | [207.0, 151.5] | [-10.692768, -8.874233] | [0.402451, -3.234386] |
Detecting your events and compute properties#
In the next step we have to detect our saccades and compute the event properties amplitude
and peak_velocity
.
We can run the microsaccade detection algorithm with its default parameters:
[6]:
dataset.detect_events('microsaccades')
20it [00:00, 25.01it/s]
[6]:
<pymovements.dataset.dataset.Dataset at 0x7f46665608b0>
Next we compute the event properties ‘amplitude’ and ‘peak velocity’ for the detected saccades.
[7]:
dataset.compute_event_properties(['amplitude', 'peak_velocity'])
20it [02:59, 8.99s/it]
[7]:
<pymovements.dataset.dataset.Dataset at 0x7f46665608b0>
Let’s verify that we have detected some saccades and have the desired columns available.
[8]:
dataset.events[0].frame.head()
[8]:
text_id | page_id | name | onset | offset | duration | amplitude | peak_velocity |
---|---|---|---|---|---|---|---|
i64 | i64 | str | i64 | i64 | i64 | f32 | f32 |
0 | 1 | "saccade" | 1988323 | 1988337 | 14 | 1.236741 | 129.856674 |
0 | 1 | "saccade" | 1988342 | 1988350 | 8 | 0.330749 | 50.527161 |
0 | 1 | "saccade" | 1988547 | 1988567 | 20 | 2.391184 | 200.144379 |
0 | 1 | "saccade" | 1988571 | 1988582 | 11 | 0.476812 | 56.048248 |
0 | 1 | "saccade" | 1988737 | 1988760 | 23 | 3.285114 | 249.678116 |
Plot the main sequence#
Now we just pass the event dataframe to the plotting function:
[9]:
# only showing the first three event dataframes here.
# note that you can adjust the styling of the plot, e.g. setting a low
# alpha value allows you to see overlapping data points
for event_df in dataset.events[:3]:
pm.plotting.main_sequence_plot(
event_df,
title='Main sequence plot for '
f'text {event_df[0, "text_id"]}, '
f'page {event_df[0, "page_id"]}',
alpha=0.3,
color='green',
marker='x',
marker_size=30,
)
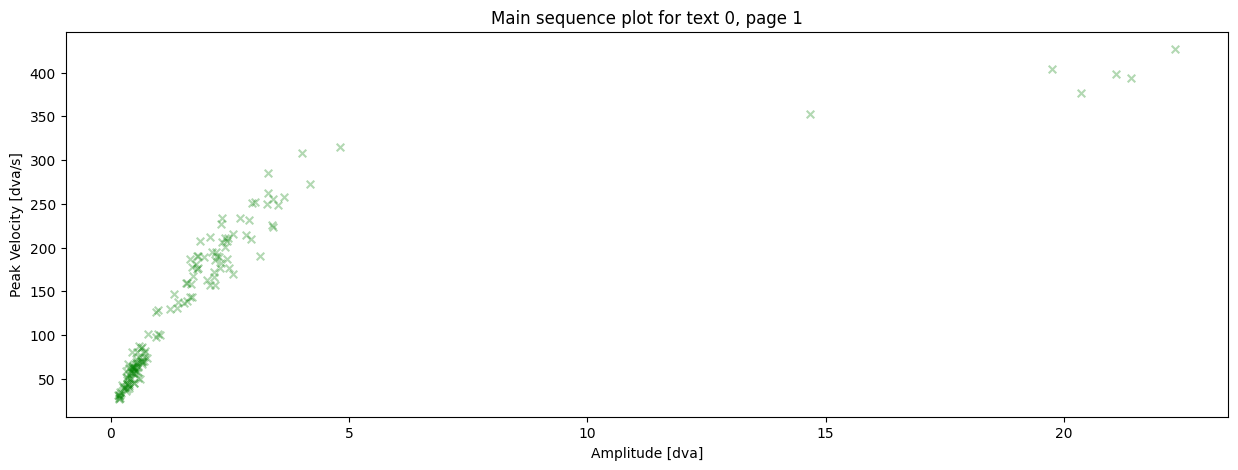
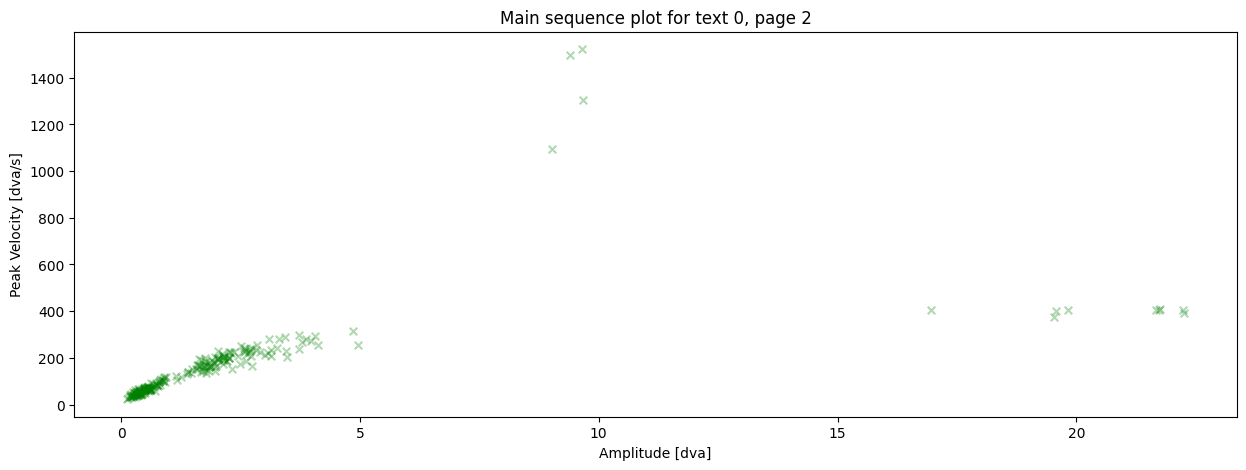
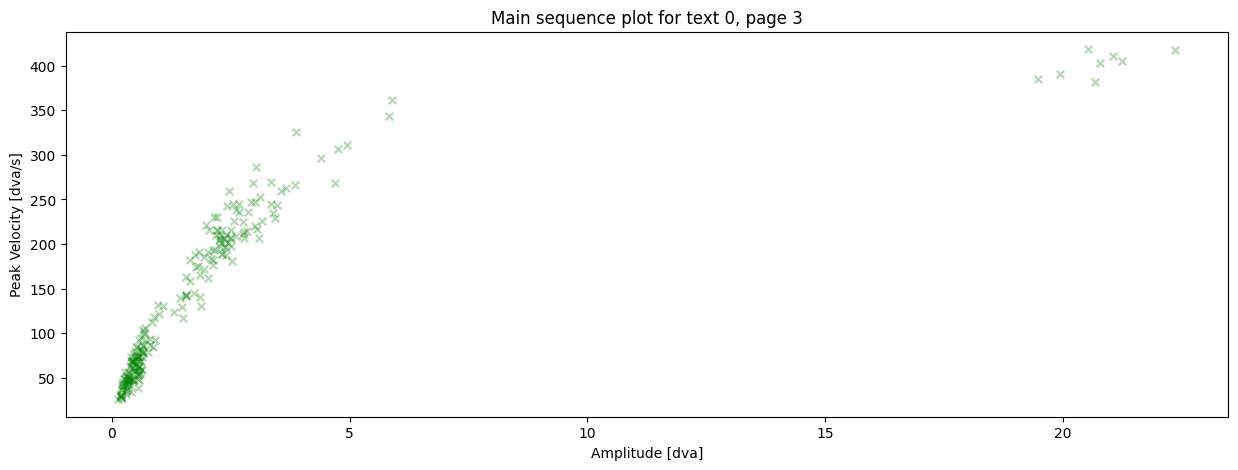
What you have learned in this tutorial:#
how to prepare your data to plot a main sequence
how to create a main sequence plot by using
main_sequence_plot